Personal tools
multimap

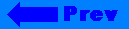
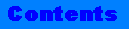
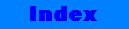
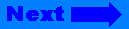
Click on the banner to return to the class reference home page.
multimap
Container
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Constructors and Destructors
- Assignment Operator
- Allocator
- Iterators
- Member Functions
- Non-member Operators
- Specialized Algorithms
- Example
- Warnings
- See Also
Summary
An associative container providing access to non-key values using keys. multimap keys are not required to be unique. A multimap supports bidirectional iterators.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Synopsis
#include <map> template <class Key, class T, class Compare = less<Key>, class Allocator = allocator<T> > class multimap ;
Description
multimap <Key ,T, Compare, Allocator> provides fast access to stored values of type T which are indexed by keys of type Key. The default operation for key comparison is the < operator. Unlike map, multimap allows insertion of duplicate keys.
multimap provides bidirectional iterators which point to an instance of pair<const Key x, T y> where x is the key and y is the stored value associated with that key. The definition of multimap provides a typedef to this pair called value_type.
The types used for both the template parameters Key and T must provide the following (where T is the type, t is a value of T and u is a const value of T):
Copy constructors - T(t) and T(u) Destructor - t.~T() Address of - &t and &u yielding T* and const T* respectively Assignment - t = a where a is a (possibly const) value of T
The type used for the Compare template parameter must satisfy the requirements for binary functions.
Interface
template <class Key, class T, class Compare = less<Key>, class Allocator = allocator<T> > class multimap { public: // types typedef Key key_type; typedef T mapped_type; typedef pair<const Key, T> value_type; typedef Compare key_compare; typedef Allocator allocator_type; typename reference; typename const_reference; typename iterator; typename const_iterator; typename size_type; typename difference_type; typename reverse_iterator; typename const_reverse_iterator; class value_compare : public binary_function<value_type, value_type, bool> { friend class multimap<Key, T, Compare, Allocator>; public : bool operator() (const value_type&, const value_type&) const; }; // Construct/Copy/Destroy explicit multimap (const Compare& = Compare(), const Allocator& = Allocator()); template <class InputIterator> multimap (InputIterator, InputIterator, const Compare& = Compare(), const Allocator& = Allocator()); multimap (const multimap<Key, T, Compare, Allocator>&); ~multimap (); multimap<Key, T, Compare, Allocator>& operator= (const multimap<Key, T, Compare, Allocator>&); // Iterators iterator begin (); const_iterator begin () const; iterator end (); const_iterator end () const; reverse_iterator rbegin (); const_reverse_iterator rbegin () const; reverse_iterator rend (); const_reverse_iterator rend () const; // Capacity bool empty () const; size_type size () const; size_type max_size () const; // Modifiers iterator insert (const value_type&); iterator insert (iterator, const value_type&); template <class InputIterator> void insert (InputIterator, InputIterator); iterator erase (iterator); size_type erase (const key_type&); iterator erase (iterator, iterator); void swap (multimap<Key, T, Compare, Allocator>&); // Observers key_compare key_comp () const; value_compare value_comp () const; // Multimap operations iterator find (const key_type&); const_iterator find (const key_type&) const; size_type count (const key_type&) const; iterator lower_bound (const key_type&); const_iterator lower_bound (const key_type&) const; iterator upper_bound (const key_type&); const_iterator upper_bound (const key_type&) const; pair<iterator, iterator> equal_range (const key_type&); pair<const_iterator, const_iterator> equal_range (const key_type&) const; }; // Non-member Operators template <class Key, class T,class Compare, class Allocator> bool operator== (const multimap<Key, T, Compare, Allocator>&, const multimap<Key, T, Compare, Allocator>&); template <class Key, class T,class Compare, class Allocator> bool operator!= (const multimap<Key, T, Compare, Allocator>&, const multimap<Key, T, Compare, Allocator>&); template <class Key, class T, class Compare, class Allocator> bool operator< (const multimap<Key, T, Compare, Allocator>&, const multimap<Key, T, Compare, Allocator>&); template <class Key, class T, class Compare, class Allocator> bool operator> (const multimap<Key, T, Compare, Allocator>&, const multimap<Key, T, Compare, Allocator>&); template <class Key, class T, class Compare, class Allocator> bool operator<= (const multimap<Key, T, Compare, Allocator>&, const multimap<Key, T, Compare, Allocator>&); template <class Key, class T, class Compare, class Allocator> bool operator>= (const multimap<Key, T, Compare, Allocator>&, const multimap<Key, T, Compare, Allocator>&); // Specialized Algorithms template <class Key, class T, class Compare, class Allocator> void swap (multimap<Key, T, Compare, Allocator>&, multimap<Key, T, Compare, Allocator>&;
Constructors and Destructors
explicit multimap(const Compare& comp = Compare(), const Allocator& alloc = Allocator());
Default constructor. Constructs an empty multimap that will use the optional relation comp to order keys and the allocator alloc for all storage management.
template <class InputIterator> multimap(InputIterator first, InputIterator last, const Compare& comp = Compare() const Allocator& alloc = Allocator());
Constructs a multimap containing values in the range [first, last). Creation of the new multimap is only guaranteed to succeed if the iterators first and last return values of type pair<class Key, class T>.
multimap(const multimap<Key, T, Compare, Allocator>& x);
Copy constructor. Creates a new multimap by copying all pairs of key and value from x.
~multimap();
The destructor. Releases any allocated memory for this multimap.
Assignment Operator
multimap<Key, T, Compare, Allocator>& operator=(const multimap<Key, T, Compare, Allocator>& x);
Replaces the contents of *this with a copy of the multimap x.
Allocator
allocator_type get_allocator() const;
Returns a copy of the allocator used by self for storage management.
Iterators
iterator begin() ;
Returns a bidirectional iterator pointing to the first element stored in the multimap. "First" is defined by the multimap's comparison operator, Compare.
const_iterator begin() const;
Returns a const_iterator pointing to the first element stored in the multimap. "First" is defined by the multimap's comparison operator, Compare.
iterator end() ;
Returns a bidirectional iterator pointing to the last element stored in the multimap, i.e. the off-the-end value.
const_iterator end() const;
Returns a const_iterator pointing to the last element stored in the multimap.
reverse_iterator rbegin() ;
Returns a reverse_iterator pointing to the first element stored in the multimap. "First" is defined by the multimap's comparison operator, Compare.
const_reverse_iterator rbegin() const;
Returns a const_reverse_iterator pointing to the first element stored in the multimap.
reverse_iterator rend() ;
Returns a reverse_iterator pointing to the last element stored in the multimap, i.e., the off-the-end value.
const_reverse_iterator rend() const;
Returns a const_reverse_iterator pointing to the last element stored in the multimap.
Member Functions
void clear();
Erases all elements from the self.
size_type count(const key_type& x) const;
Returns the number of elements in the multimap with the key value x.
bool empty() const;
Returns true if the multimap is empty, false otherwise.
pair<iterator,iterator> equal_range(const key_type& x); pair<const_iterator,const_iterator> equal_range(const key_type& x) const;
Returns the pair (lower_bound(x), upper_bound(x)).
iterator erase(iterator first, iterator last);
Providing the iterators first and last point to the same multimap and last is reachable from first, all elements in the range (first, last) will be deleted from the multimap. Returns an iterator pointing to the element following the last deleted element, or end(), if there were no elements after the deleted range.
iterator erase(iterator position);
Deletes the multimap element pointed to by the iterator position. Returns an iterator pointing to the element following the deleted element, or end(), if the deleted item was the last one in this list.
size_type erase(const key_type& x);
Deletes the elements with the key value x from the map, if any exist. Returns the number of deleted elements, or 0 otherwise.
iterator find(const key_type& x);
Searches the multimap for a pair with the key value x and returns an iterator to that pair if it is found. If such a pair is not found the value end() is returned.
const_iterator find(const key_type& x) const;
Same as find above but returns a const_iterator.
iterator insert(const value_type& x); iterator insert(iterator position, const value_type& x);
x is inserted into the multimap. A position may be supplied as a hint regarding where to do the insertion. If the insertion may be done right after position then it takes amortized constant time. Otherwise it will take O(log N) time.
template <class InputIterator> void insert(InputIterator first, InputIterator last);
Copies of each element in the range [first, last) will be inserted into the multimap. The iterators first and last must return values of type pair<T1,T2>. This operation takes approximately O(N*log(size()+N)) time.
key_compare key_comp() const;
Returns a function object capable of comparing key values using the comparison operation, Compare, of the current multimap.
iterator lower_bound(const key_type& x);
Returns an iterator to the first multimap element whose key is greater than or equal to x. If no such element exists then end() is returned.
const_iterator lower_bound(const key_type& x) const;
Same as lower_bound above but returns a const_iterator.
size_type max_size() const;
Returns the maximum possible size of the multimap.
size_type size() const;
Returns the number of elements in the multimap.
void swap(multimap<Key, T, Compare, Allocator>& x);
Swaps the contents of the multimap x with the current multimap, *this.
iterator upper_bound(const key_type& x);
Returns an iterator to the first element whose key is less than or equal to x. If no such element exists, then end() is returned.
const_iterator upper_bound(const key_type& x) const;
Same as upper_bound above but returns a const_iterator.
value_compare value_comp() const;
Returns a function object capable of comparing value_types (key,value pairs) using the comparison operation, Compare, of the current multimap.
Non-member Operators
bool operator==(const multimap<Key, T, Compare, Allocator>& x, const multimap<Key, T, Compare, Allocator>& y);
Returns true if all elements in x are element-wise equal to all elements in y, using (T::operator==). Otherwise it returns false.
bool operator!=(const multimap<Key, T, Compare, Allocator>& x, const multimap<Key, T, Compare, Allocator>& y);
Returns !(x==y).
bool operator<(const multimap<Key, T, Compare, Allocator>& x, const multimap<Key, T, Compare, Allocator>& y);
Returns true if x is lexicographically less than y. Otherwise, it returns false.
bool operator>(const multimap<Key, T, Compare, Allocator>& x, const multimap<Key, T, Compare, Allocator>& y);
Returns y < x.
bool operator<=(const multimap<Key, T, Compare, Allocator>& x, const multimap<Key, T, Compare, Allocator>& y);
Returns !(y < x).
bool operator>=(const multimap<Key, T, Compare, Allocator>& x, const multimap<Key, T, Compare, Allocator>& y);
Returns !(x < y).
Specialized Algorithms
template<class Key, class T, class Compare, class Allocator> void swap(multimap<Key, T, Compare, Allocator>& a, multimap<Key, T, Compare, Allocator>& b);
Efficiently swaps the contents of a and b.
Example
// // multimap.cpp // #include <string> #include <map> #include <iostream.h> typedef multimap<int, string, less<int> > months_type; // Print out a pair template <class First, class Second> ostream& operator<<(ostream& out, const pair<First,Second>& p) { cout << p.second << " has " << p.first << " days"; return out; } // Print out a multimap ostream& operator<<(ostream& out, months_type l) { copy(l.begin(),l.end(), ostream_iterator <months_type::value_type,char>(cout,"\n")); return out; } int main(void) { // create a multimap of months and the number of // days in the month months_type months; typedef months_type::value_type value_type; // Put the months in the multimap months.insert(value_type(31, string("January"))); months.insert(value_type(28, string("February"))); months.insert(value_type(31, string("March"))); months.insert(value_type(30, string("April"))); months.insert(value_type(31, string("May"))); months.insert(value_type(30, string("June"))); months.insert(value_type(31, string("July"))); months.insert(value_type(31, string("August"))); months.insert(value_type(30, string("September"))); months.insert(value_type(31, string("October"))); months.insert(value_type(30, string("November"))); months.insert(value_type(31, string("December"))); // print out the months cout << "All months of the year" << endl << months << endl; // Find the Months with 30 days pair<months_type::iterator,months_type::iterator> p = months.equal_range(30); // print out the 30 day months cout << endl << "Months with 30 days" << endl; copy(p.first,p.second, ostream_iterator<months_type::value_type,char>(cout,"\n")); return 0; } Output : All months of the year February has 28 days April has 30 days June has 30 days September has 30 days November has 30 days January has 31 days March has 31 days May has 31 days July has 31 days August has 31 days October has 31 days December has 31 days Months with 30 days April has 30 days June has 30 days September has 30 days November has 30 days
Warnings
Member function templates are used in all containers provided by the Standard Template Library. An example of this feature is the constructor for multimap<Key,T,Compare,Allocator> that takes two templated iterators:
template <class InputIterator> multimap (InputIterator, InputIterator, const Compare& = Compare(), const Allocator& = Allocator());
multimap also has an insert function of this type. These functions, when not restricted by compiler limitations, allow you to use any type of input iterator as arguments. For compilers that do not support this feature we provide substitute functions that allow you to use an iterator obtained from the same type of container as the one you are constructing (or calling a member function on), or you can use a pointer to the type of element you have in the container.
For example, if your compiler does not support member function templates you can construct a multimap in the following two ways:
multimap<int,int>::value_type intarray[10]; multimap<int,int> first_map(intarry, intarray + 10); multimap<int,int> second_multimap(first_multimap.begin(), first_multimap.end());
but not this way:
multimap<long,long> long_multimap(first_multimap.begin(),first_multimap.end());
since the long_multimap and first_multimap are not the same type.
Also, many compilers do not support default template arguments. If your compiler is one of these you need to always supply the Compare template argument and the Allocator template argument. For instance you'll have to write:
multimap<int, int, less<int>, allocator<int> >
instead of:
multimap<int, int>
See Also
allocator, Containers, Iterators, map
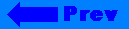
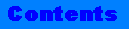
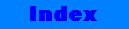
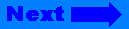
©Copyright 1996, Rogue Wave Software, Inc.