Personal tools
ptr_fun

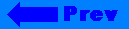
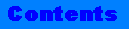
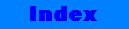
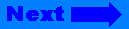
Click on the banner to return to the class reference home page.
ptr_fun
Function Adaptor
Summary
A function that is overloaded to adapt a pointer to a function to work where a function is called for.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <functional> template<class Arg, class Result> pointer_to_unary_function<Arg, Result> ptr_fun (Result (*f)(Arg)); template<class Arg1, class Arg2, class Result> pointer_to_binary_function<Arg1, Arg2, Result> ptr_fun (Result (*x)(Arg1, Arg2));
Description
The pointer_to_unary_function and pointer_to_binary_function classes encapsulate pointers to functions and provide an operator() so that the resulting object serves as a function object for the function.
The ptr_fun function is overloaded to create instances of pointer_to_unary_function or pointer_to_binary_function when provided with the appropriate pointer to a function.
Example
// // pnt2fnct.cpp // #include <functional> #include <deque> #include <vector> #include <algorithm> #include <iostream.h> //Create a function int factorial(int x) { int result = 1; for(int i = 2; i <= x; i++) result *= i; return result; } int main() { //Initialize a deque with an array of ints int init[7] = {1,2,3,4,5,6,7}; deque<int> d(init, init+7); //Create an empty vector to store the factorials vector<int> v((size_t)7); //Transform the numbers in the deque to their factorials and //store in the vector transform(d.begin(), d.end(), v.begin(), ptr_fun(factorial)); //Print the results cout << "The following numbers: " << endl << " "; copy(d.begin(),d.end(),ostream_iterator<int,char>(cout," ")); cout << endl << endl; cout << "Have the factorials: " << endl << " "; copy(v.begin(),v.end(),ostream_iterator<int,char>(cout," ")); return 0; } Output : The following numbers: 1 2 3 4 5 6 7 Have the factorials: 1 2 6 24 120 720 5040
Warning
If your compiler does not support default template parameters, you need to always supply the Allocator template argument. For instance, you will need to write :
vector<int, allocator<int> >
instead of :
vector<int>
See Also
Function Objects, pointer_to_binary_function, pointer_to_unary_function
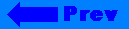
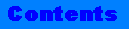
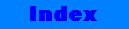
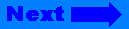
©Copyright 1996, Rogue Wave Software, Inc.