Personal tools
queue

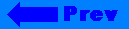
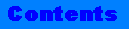
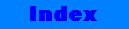
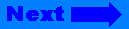
Click on the banner to return to the class reference home page.
queue
Container Adaptor
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Constructors
- Allocator
- Member Functions
- Non-member Operators
- Example
- Warnings
- See Also
Summary
A container adaptor that behaves like a queue (first in, first out).
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Member Functions | |
back() empty() front() get_allocator() operator!=() operator<() operator==() ()">operator>() pop() push() |
size() |
Synopsis
#include <queue> template <class T, class Container = deque<T> > class queue ;
Description
The queue container adaptor lets a container function as a queue. In a queue, items are pushed into the back of the container and removed from the front. The first items pushed into the queue are the first items to be popped off of the queue (first in, first out, or "FIFO").
queue can adapt any container that supports the front(), back(), push_back() and pop_front() operations. In particular, deque and list can be used.
Interface
template <class T, class Container = deque<T> > class queue { public: // typedefs typedef typename Container::value_type value_type; typedef typename Container::size_type size_type; typedef typename Container::allocator_type allocator_type; // Construct/Copy/Destroy explicit queue (const allocator_type& = allocator_type()); allocator_type get_allocator () const; // Accessors bool empty () const; size_type size () const; value_type& front (); const value_type& front () const; value_type& back (); const value_type& back () const; void push (const value_type&); void pop (); }; // Non-member Operators template <class T, class Container> bool operator== (const queue<T, Container>&, const queue<T, Container>&); template <class T, class Container> bool operator!= (const queue<T, Container>&, const queue<T, Container>&); template <class T, class Container> bool operator< (const queue<T, Container>&, const queue<T, Container>&); template <class T, class Container> bool operator> (const queue<T, Container>&, const queue<T, Container>&); template <class T, class Container> bool operator<= (const queue<T, Container>&, const queue<T, Container>&); template <class T, class Container> bool operator>= (const queue<T, Container>&, const queue<T, Container>&);
Constructors
explicit queue (const allocator_type& alloc= allocator_type());
Creates a queue of zero elements. The queue will use the allocator alloc for all storage management.
Allocator
allocator_type get_allocator () const;
Returns a copy of the allocator used by self for storage management.
Member Functions
value_type& back ();
Returns a reference to the item at the back of the queue (the last item pushed into the queue).
const value_type& back() const;
Returns a constant reference to the item at the back of the queue as a const_value_type.
bool empty () const;
Returns true if the queue is empty, otherwise false.
value_type& front ();
Returns a reference to the item at the front of the queue. This will be the first item pushed onto the queue unless pop() has been called since then.
const value_type& front () const;
Returns a constant reference to the item at the front of the queue as a const_value_type.
void pop ();
Removes the item at the front of the queue.
void push (const value_type& x);
Pushes x onto the back of the queue.
size_type size () const;
Returns the number of elements on the queue.
Non-member Operators
template <class T, class Container> bool operator== (const queue<T, Container>& x, const queue<T, Container>& y);
Equality operator. Returns true if x is the same as y.
template <class T, class Container> bool operator!= (const queue<T, Container>& x, const queue<T, Container>& y);
Inequality operator. Returns !(x==y).
template <class T, class Container> bool operator< (const queue<T, Container>& x, const queue<T, Container>& y);
Returns true if the queue defined by the elements contained in x is lexicographically less than the queue defined by the elements contained in y.
template <class T, class Container> bool operator> (const queue<T, Container>& x, const queue<T, Container>& y);
Returns y < x.
template <class T, class Container> bool operator< (const queue<T, Container>& x, const queue<T, Container>& y);
Returns !(y < x).
template <class T, class Container> bool operator< (const queue<T, Container>& x, const queue<T, Container>& y);
Returns !(x < y).
Example
// // queue.cpp // #include <queue> #include <string> #include <deque> #include <list> #include <iostream.h> int main(void) { // Make a queue using a list container queue<int, list<int>> q; // Push a couple of values on then pop them off q.push(1); q.push(2); cout << q.front() << endl; q.pop(); cout << q.front() << endl; q.pop(); // Make a queue of strings using a deque container queue<string,deque<string>> qs; // Push on a few strings then pop them back off int i; for (i = 0; i < 10; i++) { qs.push(string(i+1,'a')); cout << qs.front() << endl; } for (i = 0; i < 10; i++) { cout << qs.front() << endl; qs.pop(); } return 0; } Output : 1 2 a a a a a a a a a a a aa aaa aaaa aaaaa aaaaaa aaaaaaa aaaaaaaa aaaaaaaaa aaaaaaaaaa
Warnings
If your compiler does not support default template parameters, you must always provide a Container template parameter. For example you would not be able to write:
queue<int> var;
rather, you would have to write,
queue<int, deque<int> > var;
See Also
allocator, Containers, priority_queue
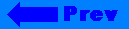
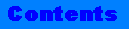
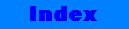
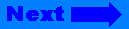
©Copyright 1996, Rogue Wave Software, Inc.