Personal tools
remove_copy_if

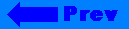
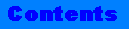
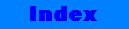
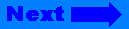
Click on the banner to return to the class reference home page.
remove_copy_if
Algorithm
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Complexity
- Example
- Warning
- See Also
Summary
Move desired elements to the front of a container, and return an iterator that describes where the sequence of desired elements ends.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class InputIterator, class OutputIterator, class Predicate> OutputIterator remove_copy_if (InputIterator first, InputIterator last, OutputIterator result, Predicate pred);
Description
The remove_copy_if algorithm copies all the elements referred to by the iterator i in the range [first, last) for which the following condition does not hold: pred(*i) == true. remove_copy_if returns the end of the resulting range. remove_copy_if is stable, that is, the relative order of the elements in the resulting range is the same as their relative order in the original range.
Complexity
Exactly last1 - first1 applications of the corresponding predicate are done.
Example
// // remove.cpp // #include <algorithm> #include <vector> #include <iterator> #include <iostream.h> template<class Arg> struct all_true : public unary_function<Arg, bool> { bool operator() (const Arg&) { return 1; } }; int main () { int arr[10] = {1,2,3,4,5,6,7,8,9,10}; vector<int> v(arr+0, arr+10); copy(v.begin(),v.end(),ostream_iterator<int,char>(cout," ")); cout << endl << endl; // // Remove the 7. // vector<int>::iterator result = remove(v.begin(), v.end(), 7); // // Delete dangling elements from the vector. // v.erase(result, v.end()); copy(v.begin(),v.end(),ostream_iterator<int,char>(cout," ")); cout << endl << endl; // // Remove everything beyond the fourth element. // result = remove_if(v.begin()+4, v.begin()+8, all_true<int>()); // // Delete dangling elements. // v.erase(result, v.end()); copy(v.begin(),v.end(),ostream_iterator<int,char>(cout," ")); cout << endl << endl; // // Now remove all 3s on output. // remove_copy(v.begin(), v.end(), ostream_iterator<int>(cout," "), 3); cout << endl << endl; // // Now remove everything satisfying predicate on output. // Should yield a NULL vector. // remove_copy_if(v.begin(), v.end(), ostream_iterator<int,char>(cout," "), all_true<int>()); return 0; } Output : 1 2 3 4 5 6 7 8 9 10 1 2 3 4 5 6 8 9 10 1 2 3 4 1 2 4
Warning
If your compiler does not support default template parameters, then you need to always supply the Allocator template argument. For instance, you will need to write :
vector<int, allocator<int> >
instead of :
vector<int>
See Also
remove, remove_if, remove_copy
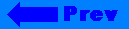
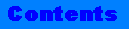
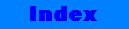
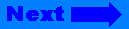
©Copyright 1996, Rogue Wave Software, Inc.