Personal tools
mem_fun, mem_fun1, mem_fun_ref, mem_fun_ref1

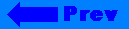
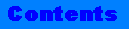
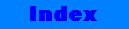
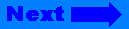
Click on the banner to return to the class reference home page.
mem_fun, mem_fun1, mem_fun_ref, mem_fun_ref1
Function Adaptors
Summary
Function objects that adapt a pointer to a member function to work where a global function is called for.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <functional> template <class S, class T> class mem_fun_t; template <class S, class T, class A> class mem_fun1_t; template <class S, class T> class mem_fun_ref_t; template <class S, class T, class A> class mem_fun1_ref_t; template<class S, class T> mem_fun_t<S,T> mem_fun(S, (T::*f)()); template<class S, class T, class A> mem_fun1_t<S,T,A> mem_fun1(S, (T::*f)(A)); template<class S, class T> mem_fun_ref_t<S,T> mem_fun_ref(S, (T::*f)()); template<class S, class T, class A> mem_fun1_ref_t<S,T,A> mem_fun1_ref(S, (T::*f)(A));
Description
The mem_fun group of templates each encapsulates a pointer to a member function. Each category of template (i.e., mem_fun, mem_fun1, mem_fun_ref, or mem_fun1_ref) provides both a class template and a function template, where the class is distinguished by the addition of _t on the end of the name to identify it as a type.
The class's constructor takes a pointer to a member function, and provides an operator() that forwards the call to that member function. In this way the resulting object serves as a global function object for that member function.
The accompanying function template simplifies the use of this facility by constructing an instance of the class on the fly.
The library provides zero and one argument adaptors for containers of pointers and containers of references (_ref). This technique can be easily extended to include adaptors for two argument functions, and so on.
Interface
template <class S, class T> class mem_fun_t : public unary_function<T*, S> { public: explicit mem_fun_t(S (T::*p)()); S operator()(T* p); }; template <class S, class T, class A> class mem_fun1_t : public binary_function<T*, A, S> { public: explicit mem_fun1_t(S (T::*p)(A)); S operator()(T* p, A x); }; template<class S, class T> mem_fun_t<S,T> mem_fun(S, (T::*f)()); template<class S, class T, class A> mem_fun1_t<S,T,A> mem_fun1(S, (T::*f)(A)); template <class S, class T> class mem_fun_ref_t : public unary_function<T, S> { public: explicit mem_fun_ref_t(S (T::*p)()); S operator()(T* p); }; template <class S, class T, class A> class mem_fun1_ref_t : public binary_function<T, A, S> { public: explicit mem_fun1_ref_t(S (T::*p)(A)); S operator()(T* p, A x); }; template<class S, class T> mem_fun_ref_t<S,T> mem_fun_ref(S, (T::*f)()); template<class S, class T, class A> mem_fun1_ref_t<S,T,A> mem_fun1_ref(S, (T::*f)(A));
Example
// // mem_fun example // #include <functional> #include <list> int main(void) { int a1[] = {2,1,5,6,4}; int a2[] = {11,4,67,3,14}; list<int> s1(a1,a1+5); list<int> s2(a2,a2+5); // Build a list of lists list<list<int>* > l; l.insert(l.begin(),s1); l.insert(l.begin(),s2); // Sort each list in the list for_each(l.begin(),l.end(),mem_fun(&list<int>::sort)); }
See Also
binary_function, Function Objects, pointer_to_unary_function, ptr_fun
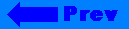
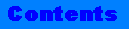
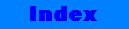
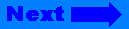
©Copyright 1996, Rogue Wave Software, Inc.