Personal tools
money_get

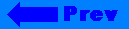
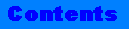
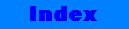
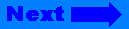
Click on the banner to return to the class reference home page.
money_get
money_getlocale::facet
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors and Destructors
- Static Members
- Public Member Functions
- Protected Member Functions
- Example
- See Also
Summary
Monetary formatting facet for input.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types | |
char_type id Intl iter_type |
string_type |
Synopsis
#include <locale> template <class charT, bool Intl = false, class InputIterator = istreambuf_iterator<charT> > class money_get;
Description
The money_get facet interprets formatted monetary string values. The Intl template parameter is used to specify locale or international formatting. If Intl is true then international formatting is used, otherwise locale formatting is used.
Interface
template <class charT, bool Intl = false, class InputIterator = istreambuf_iterator<charT> > class money_get : public locale::facet { public: typedef charT char_type; typedef InputIterator iter_type; typedef basic_string<charT> string_type; explicit money_get(size_t = 0); iter_type get(iter_type, iter_type, ios_base&, ios_base::iostate&, long double&) const; iter_type get(iter_type, iter_type, ios_base&, ios_base::iostate&, string_type&) const; static const bool intl = Intl; static locale::id id; protected: ~money_get(); // virtual virtual iter_type do_get(iter_type, iter_type, ios_base&, ios_base::iostate&, long double&) const; virtual iter_type do_get(iter_type, iter_type, ios_base&, ios_base::iostate&, string_type&) const; };
Types
char_type
Type of character upon which the facet is instantiated.
iter_type
Type of iterator used to scan the character buffer.
string_type
Type of character string passed to member functions.
Constructors and Destructors
explicit money_get(size_t refs = 0)
Construct a money_get facet. If the refs argument is 0 then destruction of the object is delegated to the locale, or locales, containing it. This allows the user to ignore lifetime management issues. On the other had, if refs is 1 then the object must be explicitly deleted; the locale will not do so. In this case, the object can be maintained across the lifetime of multiple locales.
~money_get(); // virtual and protected
Destroy the facet
Static Members
static locale::id id;
Unique identifier for this type of facet.
static const bool intl = Intl;
true if international formatting is used, false otherwise.
Public Member Functions
The public members of the money_get facet provide an interface to protected members. Each public member get has a corresponding virtual protected member do_get.
iter_type get(iter_type s, iter_type end, ios_base& f, ios_base::iostate& err, long double& units) const; iter_type get(iter_type s, iter_type end, ios_base& f, ios_base::iostate& err, string_type& digits) const;
Each of these two overloads of the public member function get calls the corresponding protected do_get function.
Protected Member Functions
virtual iter_type do_get(iter_type s, iter_type end, ios_base& f, ios_base::iostate& err, long double& units) const; virtual iter_type do_get(iter_type s, iter_type end, ios_base& f, ios_base::iostate& err, string_type& digits) const;
A monetary value is assembled
An error occurs
No more characters are available.
Digit group separators are optional. If no grouping is specified then in thousands separator characters are treated as delimiters.
If space or none are part of the format pattern in moneypunct then optional whitespace is consumed, except at the end. See the moneypunct reference section for a description of money specific formatting flags.
If iosbase::showbase is set in f.flags() then the currency symbol is optional, and if it appears after all other elements then it will not be consumed. Otherwise the currency symbol is required, and will be consumed where ever it occurs.
digits will be preceded by a '-' , or units will be negated, if the monetary value is negative.
See the moneypunct reference section for a description of money specific formatting flags.
Reads in a localized character representation of a monetary value and generates a generic representation, either as a sequence of digits or as a long double value. In either case do_get uses the smallest possible unit of currency.
Both overloads of do_get read characters from the range [s,end) until one of three things occurs:
The functions use f.flags() and the moneypunct<charT> facet from f.getloc() for formatting information to use in interpreting the sequence of characters. do_get then places a pure sequence of digits representing the monetary value in the smallest possible unit of currency into the string argument digits, or it calculates a long double value based on those digits and returns that value in units.
The following specifics apply to formatting:
The err argument will be set to iosbase::failbit if an error occurs during parsing.
Returns an iterator pointing one past the last character that is part of a valid monetary sequence.
Example
// // moneyget.cpp // #include <string> #include <sstream> int main () { using namespace std; typedef istreambuf_iterator<char,char_traits<char> > iter_type; locale loc; string buffer("$100.02"); string dest; long double ldest; ios_base::iostate state; iter_type end; // Get a money_get facet const money_get<char,false,iter_type>& mgf = #ifndef _RWSTD_NO_TEMPLATE_ON_RETURN_TYPE use_facet<money_get<char,false,iter_type> >(loc); #else use_facet(loc,(money_get<char,false,iter_type>*)0); #endif { // Build an istringstream from the buffer and construct // a beginning iterator on it. istringstream ins(buffer); iter_type begin(ins); // Get a string representation of the monetary value mgf.get(begin,end,ins,state,dest); } { // Build another istringstream from the buffer, etc. // so we have an iterator pointing to the beginning istringstream ins(buffer); iter_type begin(ins); // Get a long double representation // of the monetary value mgf.get(begin,end,ins,state,ldest); } cout << buffer << " --> " << dest << " --> " << ldest << endl; return 0; }
See Also
locale, facets, money_put, moneypunct
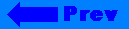
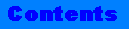
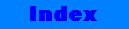
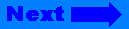
©Copyright 1996, Rogue Wave Software, Inc.