Personal tools
money_put

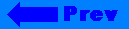
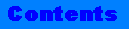
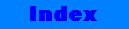
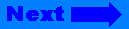
Click on the banner to return to the class reference home page.
money_put
money_putlocale::facet
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors and Destructors
- Static Members
- Public Member Functions
- Protected Member Functions
- Example
- See Also
Summary
Monetary formatting facet for output.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types | |
char_type id Intl iter_type |
string_type |
Synopsis
#include <locale> template <class charT, bool Intl = false, class OutputIterator = ostreambuf_iterator<charT> > class money_put;
Description
The money_put facet takes a long double value, or generic sequence of digits and writes out a formatted representation of the monetary value.
Interface
template <class charT, bool Intl = false, class OutputIterator = ostreambuf_iterator<charT> > class money_put : public locale::facet { public: typedef charT char_type; typedef OutputIterator iter_type; typedef basic_string<charT> string_type; explicit money_put(size_t = 0); iter_type put(iter_type, ios_base&, char_type, long double) const; iter_type put(iter_type, ios_base&, char_type, const string_type&) const; static const bool intl = Intl; static locale::id id; protected: ~money_put(); // virtual virtual iter_type do_put(iter_type, ios_base&, char_type, long double) const; virtual iter_type do_put(iter_type, ios_base&, char_type, const string_type&) const; };
Types
char_type
Type of the character upon which the facet is instantiated.
iter_type
Type of iterator used to scan the character buffer.
string_type
Type of character string passed to member functions.
Constructors and Destructors
explicit money_put(size_t refs = 0)
Construct a money_put facet. If the refs argument is 0 then destruction of the object is delegated to the locale, or locales, containing it. This allows the user to ignore lifetime management issues. On the other had, if refs is 1 then the object must be explicitly deleted; the locale will not do so. In this case, the object can be maintained across the lifetime of multiple locales.
~money_put(); // virtual and protected
Destroy the facet
Static Members
static locale::id id;
Unique identifier for this type of facet.
static const bool intl = Intl;
true if international representation, false otherwise.
Public Member Functions
The public members of the money_put facet provide an interface to protected members. Each public member put has a corresponding virtual protected member do_put.
iter_typeput(iter_type s, ios_base& f, char_type fill, long double units) const; iter_type put(iter_type s, ios_base& f, char_type fill, const string_type& digits) const;
Each of these two overloads of the public member function put simply calls the corresponding protected do_put function.
Protected Member Functions
virtual iter_typedo_put(iter_type s, ios_base& f, char_type fill, long double units) const;
Writes out a character string representation of the monetary value contained in units. Since units represents the monetary value in the smallest possible unit of currency any fractional portions of the value are ignored. f.flags() and the moneypunct<charT> facet from f.getloc() provide the formatting information.
The fill argument is used for any padding.
Returns an iterator pointing one past the last character written.
virtual iter_type do_put(iter_type s, ios_base& f, char_type fill, const string_type& digits) const;
Writes out a character string representation of the monetary contained in digits. digits represents the monetary value as a sequence of digits in the smallest possible unit of currency. do_put only looks at an optional - character and any immediately contiguous digits. f.flags() and the moneypunct<charT> facet from f.getloc() provide the formatting information.
The fill argument is used for any padding.
Returns an iterator pointing one past the last character written.
Example
// // moneyput.cpp // #include <string> #include <iostream> int main () { using namespace std; typedef ostreambuf_iterator<char,char_traits<char> > iter_type; locale loc; string buffer("10002"); long double ldval = 10002; // Construct a ostreambuf_iterator on cout iter_type begin(cout); // Get a money put facet const money_put<char,false,iter_type>& mp = #ifndef _RWSTD_NO_TEMPLATE_ON_RETURN_TYPE use_facet<money_put<char,false,iter_type> >(loc); #else use_facet(loc,(money_put<char,false,iter_type>*)0); #endif // Put out the string representation of the monetary value cout << buffer << " --> "; mp.put(begin,cout,' ',buffer); // Put out the long double representation // of the monetary value cout << endl << ldval << " --> "; mp.put(begin,cout,' ',ldval); cout << endl; return 0; }
See Also
locale, facets, money_get, moneypunct
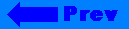
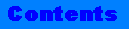
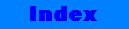
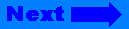
©Copyright 1996, Rogue Wave Software, Inc.